JSON Value Translator Web App
Role: Software engineer, designer
Technologies Used:
- Typescript
- Next.js
- Web Workers
- transformers.js
- Xenova/nllb-200-distilled-600M AI machine translation model
- franc natural language detector
I often need to translate sets of JSON values to multiple languages to support website localization features. Packages that use i18next
, like react-i18next
, interpret JSON files per language in this format:
1// en.json 2{ 3 "Title": "Hello, world!", 4 "Description": "A starter website" 5}
There's usually a separate JSON file for each language that has the same keys, but translated values, like this:
1// es.json 2{ 3 "Title": "¡Hola Mundo!", 4 "Description": "Un sitio web de inicio" 5}
Often, there are multiple values that I need to translate at once to multiple target languages and this is a little tedious to do by hand using tools like Google Translate. So, I made the JSON Value Translator tool that can handle translating all values in a one-level deep JSON file to multiple languages and lets you download the separate JSON files per target language.
While Google Translate has an API that can be used to programmatically generate translations, I decided to use AI for the task for two reasons:
- Cost - it's free to use open-source in-browser AI models, but there's a cost to calling the Google Translate API
- Learning opportunity - I wanted to learn from building a web app that interacts with an in-browser AI model
AI Models
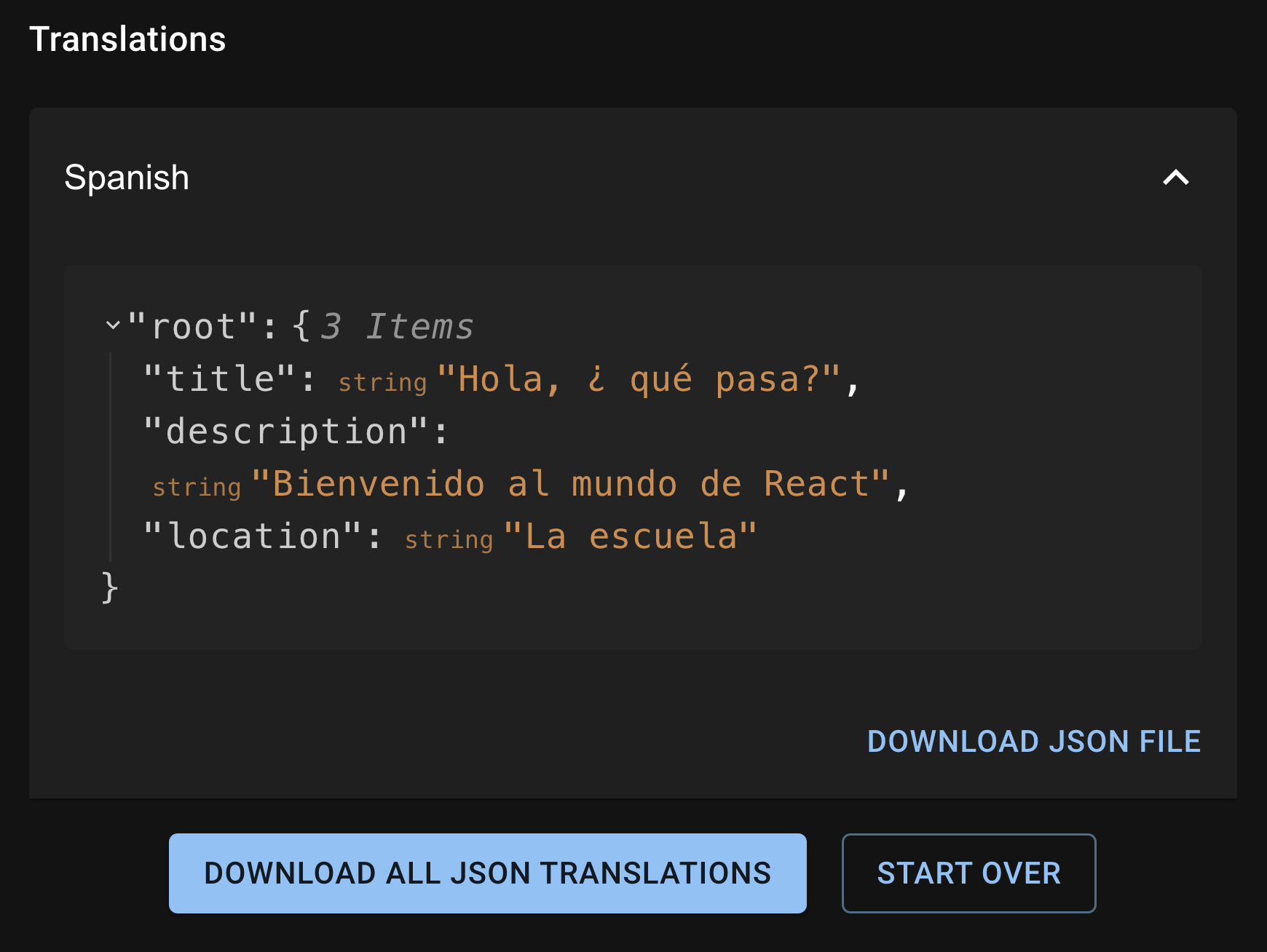
franc for Natural Language Detection
The web app attempts to detect the initial language by analyzing the uploaded JSON file with the franc
library. It's not very accurate for small amounts of text, so it tends to perform better if you're translated a larger set of JSON values. The model used by the app currently supports 187 languages. If franc
gets the language wrong, though, users can select the correct language.
Xenova/nllb-200-distilled-600M AI machine translation model for Translations
The translations are handled by the Xenova/nllb-200-distilled-600M
AI Large Language Model (LLM), which supports 200 languages. The web app runs the model in the browser in a Web Worker through the transformers.js
library. It takes some time to initialize the model the first time you load the page and running it in a Web Worker takes that work off the main thread, allowing users to continue to interact with the web app as the model loads in the background.
In using the model to generate translations, I've noticed that it generally seems accurate, but it does take some creative liberties. When translating "Hello" to Spanish, for example, it returns "Hola, ¿que pasa?" instead of just "Hola". Since it is an AI model, users should still double check the translations returned to make sure they seem accurate. In a future iteration, I'd like to explore using other models that allow for additional optimization parameters to reduce the model's "creativity".